Gravity Forms Tag Editor
A simple plugin that makes modifying Gravity Forms tags a breeze. Change any attribute of the form tag with just a few lines of code.
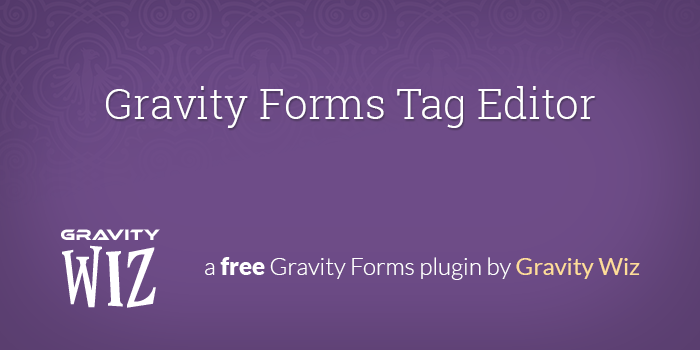
September 14, 2016: Added support for {formId} merge tag for use in property values.
Getting Started
- Make sure you have Gravity Forms installed and activated.
- Click the “Download Code” button above and save the file to your Desktop.
- Drop the file into your WordPress plugins folder via FTP – or – zip the file up and upload it via WordPress plugin uploader.
You’re now ready to configure the plugin.
Plugin Configuration
This is plugin does not provide a user interface. You will need to configure it’s functionality via small code snippets that can be copy-and-pasted into your theme’s functions.php file.
This plugin uses the gform_form_tag filter to convert the form tag string into an array of properties which can then be easily modified, removed, or added.
Here is an example of a basic configuration. This example will modify the “action” parameter to post the form to 3rd party URL instead of the default action URL.
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'form_id' => 123,
'action' => 'https://mythirdpartyservice.com',
) );
}
To apply a configuration to all forms, simply do not specify a form_id
parameter.
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'action' => 'https://mythirdpartyservice.com',
) );
}
Parameters
There are only two default parameters.
tag string (required)
Specify the Gravity Forms HTML tag that should be modified. Currently only ‘form’ is supported.
form_id integer (optional)
Specify a form ID to which the custom tag attributes should be applied. To apply the properties to all form tags, exclude this parameter.
All other parameters will automatically be added as attributes to the specified tag. See the usage examples below to see how any attribute can be added, removed, or modified.
Usage Examples
Disable HTML5 Autocomplete
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'autocomplete' => 'off',
) );
}
Add Custom Event Attributes
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'form_id' => 123,
'oninput' => 'function() { alert ( "Something has been input!" ); }'
) );
}
Add a Name Attribute
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'name' => 'form_{formId}',
) );
}
Disable HTML5 Validation
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'novalidate' => 'novalidate'
) );
}
For more information on disabling HTML5 validation see our article on the subject.
Process Form via a Custom Script (by modifying the action attribute)
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'form_id' => 123,
'action' => '/path/to/my-custom-form-handler.php'
) );
}
Add Custom Data Attributes
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'form_id' => 123,
'data-persist' => 'garlic'
) );
}
Garlic.js is a Javascript library which allows your form data to persist locally to prevent accidental form data loss.
Submit Form via Get Method (by changing the method attribute)
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'form_id' => 123,
'method' => 'get'
) );
}
Modify the Target Attribute
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'form_id' => 123,
'target' => '_parent'
) );
}
This is useful if you’re loading a Gravity Form in an <iframe>
based modal but want to redirect on the parent page and not within the modal <iframe>
.
Submit Form to a New Tab/Page (by modifying the target attribute)
if ( class_exists ( 'GW_Tag_Editor' ) ) {
new GW_Tag_Editor( array(
'tag' => 'form',
'form_id' => 123,
'target' => '_blank'
) );
}
Any questions?
We’d love to to hear your feedback on this bare bones plugin. Did it make your life any easier? Do you have any features on your wishlist? Share your thoughts with us in the comments below!
Are any merge tags available apart from {formId}? I’m doing some GA4 integrations, and it can capture these parameters: form_id: HTML id attribute of the
<
form> DOM element it grabs #id successfully form_name: HTML name attribute of the
<
form> DOM element form_destination: URL to which the form is being submitted it concatenates current URL and action (for AJAX at least) form_submit_text: text of the submit button, if present currently it’s not autoatically grabbing this – a problem for another day.
So it’s just ‘name=’ missing. Can this merge tag be added?
Hey Hamish, it looks like your message formatting got a little jumbled up and I’m not sure if I’m understanding your question. Could you clarify what you’re trying to achieve here?
Hello Support,
When using target => _blank, is there a way to prevent the submit button from opening a new tab until all the required fields are filled out? Right now, if you click submit without filling anything it still opens a new tab. You can see this on my site. Thanks
if( class_exists( ‘GW_Tag_Editor’ ) ) { new GW_Tag_Editor( array( ‘tag’ => ‘form’, ‘form_id’ => 3, ‘target’ => ‘_blank’ ) ); }
Thanks for the question, Robert. For other folks reading this, we created a ticket for Robert and have been exploring what is possible.
Hello, can you list several items on one script such as:
if( class_exists( ‘GW_Tag_Editor’ ) ) { new GW_Tag_Editor( array( ‘tag’ => ‘form’, ‘form_id’ => 123, ‘target’ => ‘_blank’ ‘oninput’ => ‘function() { alert( “Something has been input!” ); }’ ) ); }
Hi Robert,
I’m not sure if I understand you correctly, because the example you have there is a usage example to Add Custom Event Attributes. How are you looking to list several items on one script? That said, note that whatever script is within the anonymous function will run oninput.
Best,
how do you apply the form action when the form has two pages?my problem right now is that the next button has the same “ID” so it automatically send when next button is press
Not sure I understand your question?
Hi There, A question on changing multiple forms with different ID’s, would you add multiple if statements or would you combine them?
Hi Justin, this will help: https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
Hi, more easy with one example… see the code in input > class please
Thanks
Currently only the form tag is supported.
Great stuff!
But if i need add
<
form class=""> ?
And where i put these cod?
This might help: https://gravitywiz.com/documentation/snippet-troubleshooting/
[…] Plugin: Gravity Forms Tag Editor […]
Thanks for sharing this tool! Wondering if you can direct me with it… I am trying to have a confirmation redirect URL open in another tab. (Using to pass through to a reservations site). So the gravity form is correctly creating the redirect custom query string, but I’d like that redirect URL to open with “_blank”. Is there a tweak I can do to make that happen? Thank you!!!
Hm, I don’t know of an existing solution for this. The simplest approach would probably be to use a text-based confirmation and write a bit of JS to automatically load the new window with the desired URL when the confirmation loads.
Thanks David for the insight! I appreciate the quick reply.
What if I only want to process particular fields within each form to a special handler, everything else just goes to the original.
Hi Nick, you’d probably want to do this after the submission via the gform_after_submission action. You can pull the specific field values you want out of the $entry object and post them via wp_remote_post() to your special handler.
Modify the Target Attribute.
If we are using Multi Step form, after code inserted page redirect on first step itself. We need to target _parent or _blank after form submitted on final or last step of “Multi Step Form”.
How can we achieve that with this AWESOME code snippet?
Please help on this.
Thanks!
You would want to only init the code if the last page is being rendered. Here’s an example: http://snippi.com/s/1mqjtm4
Thank you David for your help.
I used another way: redirect page reload again on _parent. But your solution is correct.
Thanks again!
-Vinu Narayanan
Awesome. Glad you were able to find a solution. :)
Hi. In using the following snippet:
if( class_exists( ‘GW_Tag_Editor’ ) ) { new GW_Tag_Editor( array( ‘tag’ => ‘form’, ‘name’ => ‘form_{formId}’, ) ); }
being enclosed in ‘ ‘, {formId} would just literally form part of the string. for it to be more useful though, ideally {formId} will be replaced with the value of the corresponding form Id. What’s the correct way of dynamically replacing the value of the {formId}?
Hi PJ, I’ve added support for the {formId} merge tag. Download the latest version above. :)
Do we need to download garlicjs or is it included in the package?
This snippet doesn’t use garlicjs. Happy to clarify if there is any confusion.