How to Populate and Modify Dates and Times with Gravity Forms
Populate a Date or Time field with a modified value based on the current date/time or a user-submitted date/time.
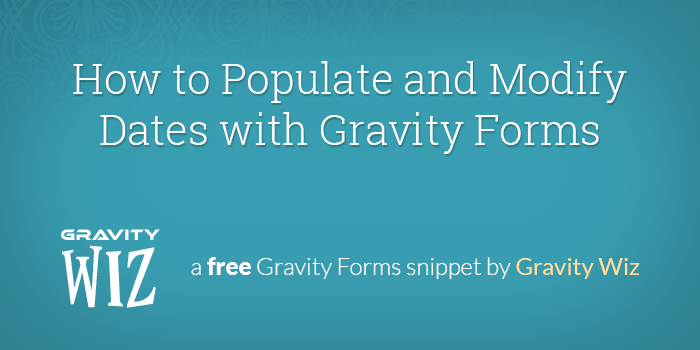
December 11, 2023: Fixed compatibility issue with Gravity Forms 2.8.
September 20, 2022: Added support for modifying dates by a number of weekdays via the "weekday" and "weekdays" keywords.
Way back when (in 2012), we wrote an article on how to populate a date, one year from the current date. We’ve rewritten this snippet to be more flexible, easier to configure, and added support for creating a Gravity Forms auto populate date setup based on a user-specified date.
What does that mean? It means you can let the user select a date via a Date field and then you can add a year to that date and populate it into another field.
How is that useful? Maybe you’re selling a year-long membership where the user can define when they would like their membership to begin. They select the date they would like their membership to begin (via a Gravity Forms Date field) and this snippet would allow you to populate the date their membership would expire (a year later).
Why would you need the expiration date? Well, first of all… you ask a lot of questions. But seriously, it’d be nice if you could let the user know when their membership expires in the confirmation message or a notification email. If you’re super pro, you could even automatically cancel the membership after the expiration date (you’d need some extra code to do this).
This is just one example of how this snippet might be used. I’m sure there are a million more and I want to hear about them. Make sure you share your usage ideas in the comments.
This snippet now supports populating Time fields! Need to let the user know an exact time their rental needs to be returned? Capture the current time, add 24 hours, and show the user the return time. 🧙🏻♂️
Getting Started
Check requirements
- Make sure you have Gravity Forms installed and activated.
- Already have a license? Download Latest Gravity Forms
- Need a license? Buy Gravity Forms
- Make sure you have Gravity Forms installed and activated.
Install the snippet
- Copy and paste the entire snippet into your theme’s functions.php file.
Configure the snippet
- At a minimum, you will want to set the
form_id
parameter to the ID of your form and thetarget_field_id
to the ID of the field in which the date should be populated. If you’d like to modify the date to be populated, set themodifier
parameter with a value like+1 year
or+7 days
. - There are many, many more configuration options available for this snippet. Continue reading if your specific need has not already been covered.
- At a minimum, you will want to set the
Gravity Forms Auto Populate Date Usage Examples
Populate Current Date (Today)
new GW_Populate_Date( array(
'form_id' => 39,
'target_field_id' => 4,
) );
Populate a Date field (or any other text-based field type) with the current date by replacing the form_id
parameter with your Gravity Forms form ID and the target_field_id
with the ID of the field you want to be populated.
If you’d like to make this date visible to the users but not editable, you can set any field to “Read Only” using the GP Read Only plugin. Just check the “Read Only” option in the field settings and users will be able to see the field value but not modify it.
Populate Date One Day from Today
new GW_Populate_Date( array(
'form_id' => 39,
'target_field_id' => 6,
'modifier' => '+1 day'
) );
Set the modifier
parameter to modify the current date. You can add or subtract time and in some really neat ways. Additional usage instructions below.
Populate Current Time (Now)
new GW_Populate_Date( array(
'form_id' => 39,
'target_field_id' => 4,
) );
Populate a Time field with the current time by replacing the form_id
parameter with your Gravity Forms form ID and the target_field_id
with the ID of the field you want to be populated.
Populate Time One Hour from Now
new GW_Populate_Date( array(
'form_id' => 39,
'target_field_id' => 6,
'modifier' => '+1 hour'
) );
Set the modifier
parameter to modify the current time. You can add or subtract time and in some really neat ways. Additional usage instructions below.
Populate Date One Year from Today with a Custom Date Format
new GW_Populate_Date( array(
'form_id' => 39,
'target_field_id' => 7,
'modifier' => '+1 year',
'format' => 'F j, Y' // i.e. March 10, 2015
) );
Set the format
parameter if you’d like to populate the date in a specific format. In this example, the date would be output like so: “September 20, 2014”. The default format is Y-m-d
which would look like this: “2014-09-20”. Additional usage instructions below
Populate Date One Year from User-specified Date
new GW_Populate_Date( array(
'form_id' => 39,
'target_field_id' => 3,
'source_field_id' => 1,
'modifier' => '+1 year'
) );
Set the source_field_id
parameter to the ID of the field from which the submitted value should be modified and used to populate the target field. The source field will generally be a Date field where the user can select a date.
Modify Date by Field Value
new GW_Populate_Date( array(
'form_id' => 1895,
'target_field_id' => 2,
'source_field_id' => 1,
'modifier' => array(
'type' => 'field',
'inputId' => 3,
'modifier' => '+{0} days',
),
) );
The modifier
parameter accepts an array which is used to modify the date by field value. Set the inputId
parameter to the ID of the field that should modify the target field value. The modifier field should be a Number field for user input. The modifier
inside the array behaves the same as other use cases, except {0} will be replaced with the field value.
The modifier
parameter also works with Time fields.
Force Minimum Date
new GW_Populate_Date( array(
'form_id' => 828,
'target_field_id' => 2,
'modifier' => '+1 year',
'min_date' => '07/16/2022',
) );
Set the min_date
parameter to force a minimum date until the modifier exceeds it.
Format Date in Specific Language
setlocale( LC_TIME, 'es_ES' );
new GW_Populate_Date( array(
'form_id' => 144,
'target_field_id' => 2,
'modifier' => '+7 days',
'format' => '%A',
'enable_i18n' => true,
) );
Use setlocale to specify the locale and set the enable_i18n
parameter to true. The target field will output the date using the chosen language.
Parameters
Here is a full list of the available parameters and additional information on how each can be configured.
form_id (integer) (required)
The ID of the form.
target_field_id (integer) (required)
The ID of the field that you want to populate with the current date.
-
The format in which the date should be populated into the target field. Default value:
Y-m-d
(i.e. “2014-09-20”). Refer to the PHP date() function for a full list of available date formatting options.If your target field is a Date field, the populated date will be formatted using whatever date format is selected in the field settings.
source_field_id (integer) (optional)
The ID of the field whose submitted value will be modified and populated into the target field.
-
A time specific string that will be used to modify the date of the target field.
Refer to the PHP Relative Formats doc for a full list of available date modification commands. Some examples include:
+1 hour
,+1 day
,+2 weeks
,+5 weekdays
,next Thursday
, andlast Monday
. min_date (integer|string) (optional)
A timestamp or date string (i.e. ’01/01/2016′) that will enforce a minimum calculated date when modifying a user-specified date.
This is particularly useful when calculating a renewal date when the user is renewing in advance of their subscription end date.
enable_i18n (boolean) (optional)
Format date and time according to locale. Locale must be set using setlocale.
override_on_submission (boolean) (optional)
Set to true to repopulate data on submission, overriding the pre-rendered value. This is useful if want the date to be based on the time of submission rather than the time the form loads.
How’d we do?
If you use it and like it, let us know. We’d love to hear the different ways you found this code useful!
I keep getting this error… irs not working
Something went wrong. Your change may not have been saved. Please try again. There is also a chance that you may need to manually fix and upload the file over FTP.
Hi Octavia,
I’m guessing you’re not adding snippet properly to your function.php file. Please refer to our documentation for details on how to install a snippet. https://gravitywiz.com/documentation/how-do-i-install-a-snippet/
I hope this helps.
Best,
Hi Can you dynamically populate the date field from url parameters? I have url parameters for day, month and year, which I’d like to use to populate the date field. Thanks Ian
Hi Ian,
You can use the Graivity Forms Dynamic population setting to populate the field with the date value passed via the query parameter.
Best,
Hi. This looks very interesting to apply. However when playing around with the snippet, I noticed that if a modifier is used which changes the transition period from AM to PM (or vice versa), it displays incorrectly. For example, using a time of 12:15pm with a modifier of -1 hour, it gives me a result of 11:15pm and not the expected 11:15AM. Is this a known issue?
Hi Peter,
I was able to recreate the issue. It appears the snippet works well if the Time Field format is set to 24 hours. I will pass this over to our developers to see if support for 12 hours Time fields can be added. We’ll update the comment when we have more information.
Best,
Hi Peter,
This issue has been fixed. Please download the latest version of the code to get it working for you.
Best,
Hello! Please, tell me what wrong: new GW_Populate_Date( array( ‘form_id’ => 10, ‘source_field_id’ => 2, ‘target_field_id’ => 6, ‘modifier’ => ‘first Tuesday’, ‘format’ => ‘d.m.Y’, ) );
I tried to get a first Tuesday of month for source field, but I get first Tuesday for chosen week (not month)
When “first Tuesday of” I always get 01.01.1970
Hi,
This doesn’t appear to be currently supported. If you have an active Gravity Perks Pro license, you can get in touch via our support form and we can get our developers to add support for this.
Best,
I keep getting invalid date
I have the config as
new GW_Populate_Date( array( ‘form_id’ => 1, ‘source_field_id’ => 5, ‘target_field_id’ => 6, ‘modifier’ => ‘+7 days’ ) );
Hi JS,
The configuration seems correct. This will require digging into your code to see what could be going on. If you have a Gravity Perks license, can you get in touch with us via our support form?
Cheers,
is it compatible with the date merge tags snippet?
other than that i dont have anything else custom
Hi JS,
They should work together the same as with all of our Perks and Snippets. That being said, a specific configuration on your site or form could be conflicting in this case. That will require some digging. You can try deactivating one of the Snippets and see if that fixes the issue.
Best,
Great and details article.
But what if I want to display the current date and increase it by 2. For example, today’s June 01, 2022, what if I want to display June 03, 2022 instead.
I also notice when I put [eid tag=”{today}” /] it gives me the current date but I’m not able to display the date increase by 2. How can I do this, please?
Ps: I’m using eid tag because I’m using elementor
Hi Pedro,
The {today} merge tag isn’t a functionality of the snippet used in this tutorial. It’s part of Gravity Forms Core. However, using the snippet you can populate a Date field with the current date increased by 2 but I am not sure that will work with a Merge tag.
You’ll need some custom code to get a merge tag to display the current date increased by 2 days. If you have an active Gravity Perks license, you can get in touch with us via our support form so we can look into this further.
Best,
So forgive me for asking likely a simple question. I don’t need to use this everywhere, but I do need to use it on two different forms with different form, source and targets for each.
I can’t call GW_Populate_Date more than once in a snippet, and this does not seem to work with the usual GW_Populate_Date_4 (form id) notation.
Suggestions on using this specifically for two different forms?
Not need to apologize! You can instantiate the class multiple times and specify the form ID inside of each class.
Thank you Dario for clarifying. I think a workaround could be using a hidden field to convert the 24hour time to a 12 hour time format. However I am not sure how to do this. Can you advise?
Thanks!
Hi Will,
You could use Copy Cat perk to copy the values from one field to another.
Best,
Hi. Interesting solution. But it isn’t about copying values. It is the format. For example if i have “15:00” to convert, it would not convert to 3:00 pm. What I understand of Copy Cat is that it copies values exactly from the source field. Am I correct?
Hi Will,
Yes, Copy Cat will copy the value as it is. If you have coding skills you could filter the value to get a calculated hour based on the input field. Otherwise, I would suggest reaching out if you have a Gravity Perks license via the support form so we can assist you further with this.
Cheers,
This is great! I am able to add e.g. 1 hour to a time field (12 hour clock), but cannot get it to work if the source field is a 24 hour clock. Can you help?
Hi Will,
This is a known issue. The snippet currently only works with Time fields set to 12 Hours. We don’t have an ETA about when this functionality will be added.
We hope to have a solution soon 🧙♂️
Best,
Hi I have a date field in Jalali (= persian, farsi, shamsi) format. It works fine. I have an another date field and want to populate it’s value based on that Jalali date field in Gregorian format.
I try this snippet and it works well.
But It can not convert the date, Just copy the first one and paste it in second one.
Hi Mo,
We’ll need more information about your setup and configuration to be able to assist you. If you have a Gravity Forms License, you can get in touch with us via our support form with an export of your form and any additional information so we can assist you further.
Best,
Is it possible to make the modifier dynamic by linking it to a gravity form field? For example, if the user selects 5 the auto-populated date would be 5 days in the future?
Hi,
From what I understand, this is currently not supported.
If you have an active Gravity Perks License, you can get in touch with us via our support form with your account email address and we’ll be happy to dig into this further.
Best,
I have multiple forms – I use the same field id but different form id’s. I would like to configure the snippet so it can work with multiple forms, is there a way to indicate several form id’s in the new gw-populate-date function?
Nevermind, I found the answer. => https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
Thanks!
Hi Edward,
Awesome, glad you were able to find your answer in that documentation.
Best,
Hi, Is it possible to apply this for a particular step of Gravity Flow? In the start form, a field called number of days is filled out. I have to add that field to a field of type date in a particular step of Gravity Flow. How can I do this?
If the step is a User Input step, then yes. The snippet will only trigger when a user is interacting with the form. If the Gravity Flow step is of another type, that isn’t supported.
I’m having a strange issue. When I see filed created by this snippet on a form it looks ok BUT when it’s actually submitted/stored, an entry has a strange fixed date and time that was not set for every single entry, no matter when it is submitted at later times.
Example of what I’m using:
setlocale( LC_TIME, ‘sl_SI.UTF-8’ );
new GW_Populate_Date( array( ‘form_id’ => 1, ‘target_field_id’ => 23, ‘modifier’ => ‘+28 hours’, ‘format’ => ‘l, j. F 0B %k:i’, ‘enable_i18n’ => true, ) );
It’s even stranger, because when I tested it, it worked OK and the next user submission after that on a live page as well, but on all the next ones the above happens.
What could be the issue here?
Thanks!
Hi Jernej,
This will be a bit difficult to determine the actual cause of the issue without extensive troubleshooting. If you have a Gravity Perks license, you can get in touch with us via our support form and we’ll be happy to dig into this further.
Best,
Can I use this to get a long date format for a date picker field? I would like to get a mergetag showing January 1, 2021 instead of 1-1-2021. Can I do this directly on the same field or do I need to add this into a new field? Thanks
Hi Mauricio,
Populating a Date field with the long date format won’t work, because it won’t allow you to submit the Date in that format. Instead of using the Date field, you can populate the Date in the long format into a Single Line Text field and this should work for you. Refer to the PHP date() function for a full list of available date formatting options.
Best,
Dear Team,
How can I populate +1 year to the end date of that month from the current date?
Hi Raja,
I’m not sure I understand your question correctly, but what I am getting is that you want to populate a Date field with a date 1 year from the current date. If so then you can use a snippet configuration with the modifier set to +1 year similar to what’s below, to do that;
new GW_Populate_Date( array( 'form_id' => 39, 'target_field_id' => 6, 'modifier' => '+1 year' ) );
Best,
Hello,
Thanks for this feature.
I have one specific case. Maybe you can help me with that ?
I want to change the modifier parameter according to the value of a specific field.
If the user select the value “3”, the modifier parameter will be “+3days”, else if the user select the other value the modifier parameter will change accordingly.
How can it be done ? Thank you
I think I found the answer up there…
Hi Paul,
Glad you were able to figure it out.
Best,
Can this be used on multiple forms? Do you just copy/paste the array for each form you want it used on?
Hi Justin,
You can use this on multiple forms by creating multiple instances of the snippet’s Class. Below is the link to the documentation on how to do that;
https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
Best,
Hi, I have this installed and it works to set a default date two days in the future, but it does not block out the dates up to then. How do I set it so that the user cannot select any date less than two days from now?
I restricted the dates with this script:
http://snippi.com/s/jtrss4a
Hi Mark,
This can be accomplished with GF Limit Dates.
This may be just what we need. I work for made-to-order sauna manufacturer. During this Covid-19 pandemic, demand for saunas surged 300%. Thusly … they’d love to create the following functionality
Probably not possible with gravitywiz, but I’ll ask… * Automatically Put this date on the staffs’ calendar. Drag’n’drop on an actual calendar would be really amazing since dates need to be moved. Moving a date updates the production date field.
Hi Dan,
This is a specific use case and I am not really sure you can achieve everything using Gravity Forms. That said, you could definitely use the snippet in this tutorial to populate another date field with the production date. You can then use GP Populate Anything to populate a field with the category ID of the selected Product, which will be used to determine if the Product is Suana Build or not.
Regarding your request to have a drag and drop feature within a calendar, unfortunately, we do not have a solution to this. You may want to speak to a developer to see if they can assist with ideas or solutions to this. You can hire a developer from Codeable.io
Best,
Could we take the date from the submission of the entry and add one day onto that? I am running into an issue where a form was submitted at 8:19 am CST on 12/23/20 but the field that is storing the next business day is listed as 12/23/20 when it should be 12/24/20, so I think I am running into a timezone issue. So, if I could just use the submission entry date and add a weekday onto that things should be fine.
Hi Michael,
Currently specifying the time zone in the snippet isn’t supported. It matches your site’s time zone, which is set within the WordPress settings. You’ll find it in the General settings.
Scott,
Thanks for the reply.
If this is the case why could it be that that on a form was submitted at 8:19 am CST on 12/23/20 but the field that is storing the next business day was listed as 12/23/20 when it should be 12/24/20?
Hi Michael,
If the server time zone is set correctly, it sounds like there’s something else at play. If you have a Gravity Perks license, drop us a line and we’ll be happy to dig into this for you.
Thank you for the information. Another question – Is it possible to specify the timezone in this snippet?
Hey Michael, this is an interesting question. What is your intention behind changing the timzeone? Let us know so we could help you out with one.
Ryan,
A form on my website was submitted at 8:19 am CST on 12/23/20 but the field that is storing the next business day is listed as 12/23/20 when it should be 12/24/20. Another entry was submitted at 9:27 am CST on 12/23/20 and the field that is storing the next business day is correct. So, I think I am running into a timezone issue. That was my intention for specifying a timezone to make sure the next business day’s date is correct.
Thank you for the help! Michael
Hello!
Thank you for this! Would it be possible to calculate +1 Business Day from the Current date? For example if the form was submitted Monday-Thursday the date calculated date would be the next day. However if the form was submitted Friday-Sunday the date calculated would be the following Monday.
Hello Michael, You could actually pass the +1 weekdays modifier to allow for the days only count Monday – Friday.
Hi Ryan, trying to create +2 day Monday to Friday only, no weekends. ‘modifier’ => ‘+1 weekdays’, not working, can you help? So the idea is, populate a date +2 days, Monday to Friday only, so Thursday should be Monday, and Friday should be Tuesday. Thanks.
Hi Pavel,
Since you already have a ticket with the same issue, we’ll continue assisting you via the ticket, so that we can keep track of this properly.
Best,
thanks,this is great! Can I ask: does this account for leapyears? E.g if you want to populate a date 1year ahead in a leapyear, how would you use this?thanks!!
Hello Daniel, since this is using the PHP date function, the PHP’s DateTime class does support leap years.😀
Hello,
I have added this code and it works great. I looked through the comments and could not find how do you add more than one ID and Field ID number?
Do I keep repeating the code or can I just add it to the end of this code new GW_Populate_Date( array( ‘form_id’ => 3, ‘target_field_id’ => 158, ) );
I tried all kinds of ways by: ‘form_id’ => 3, 13, ‘form_id’ => 3, ‘form_id’ => 13, repeating the complete code up above and none of this worked.
So how do add another ID and Field ID without having to repeat the code over and over and over.
Thank you so much!!
P.S. What plugin did you use to add “Notify me of followup comments via e-mail” down below. We would like to add this to our website. We are currently using GeneratePress. Sorry I know this has nothing to do with the topic but I thought it could not hurt to ask.
Hi Brandon,
You can instantiate the class multiple times to use the snippet with multiple forms. More info here:
https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
We’re using this plugin for comment subscriptions.
Is there a way to save a date entry from Gravity Forms as a timestamp rather than as a date/time format? A user selects a date , or a date/time , as normal using the date picker , but then the value is saved as a timestamp? Thanks
Hey Chris, Yes, Gravity Forms includes the timestamp for when the form was submitted. It is an available form field when exporting entries, etc. It is called Entry Date. Let us know if you need anything else.
Thanks for the reply. I’m not looking for the ‘entry date’ as a timestamp, but rather a date field that a user selects a date, or date/time. There are several formats for saving a date in GF, but none of them are TIMESTAMP. Just wondering if there is a way to automate a field populating with the timestamp format of a date that a user selects?
Hi Chris, As you rightly said GF date fields do not support TimeStamp, but with some custom JS snippet you could populate a single-line text field with the timestamp of a date selected by a user. If you have a Gravity Perks License, you can drop us a line via the support form so we can assist you with this.
Best,
Perfect, thanks .
Hi,
I need one single Date field that is populated by today’s date + a user specified number of weeks. I have a dropdown menu where the user selects their “due date”, and the options they have is 1, 2, 3 or 4 weeks.
So the date field would be populated with todays date + 1, 2, 3, or 4 weeks – depending on what the user selects from the dropdown.
I’ve tried using the Date Time Calculator, but can’t seem to get it to work.
I can use the snippet on this page to get 4 different date fields populated with the right dates, and use conditional logic to display the correct date, but I need 1 single date field that is the final output for the “due date”.
Hope that makes sense.
Any ideas how I can do this?
Cheers Ian
Hi Ian,
This looks like something that can be done with some customization to the snippet. If you’re a Gravity Perks customer, can you please drop us a message via the support form and we’ll be happy to assist you. Remember to include an export file of the form.
Best,
Hi,
I am trying to work with GP Date Time Calculator to :
How can I make that work?
Hello Tobias, Excellent question. You will need to create multiple instances of the same snippet. More details here: https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/.😃
Hi,
i did initially try with the date class snippet here, but wanted to just get it to work natively with your date time plugin.
I do have this partially working, but not with the desired output. Her ethe example I have (after the function, but seems I can get rid of that due to having the paid plugin)
Here is what I have :
Working:
new GW_Populate_Date( array( ‘form_id’ => 1, ‘target_field_id’ => 29, ‘modifier’ => ‘+1 day’ ) );
Not working as intended $difference = $entry[’52:value’];
new GW_Populate_Date( array( ‘form_id’ => 1, ‘target_field_id’ => 53, ‘modifier’ => ‘$difference’ ) );
So that difference field just contains : Age Label value 1 -1 year 2 -2 years
So I am trying to dynamically deduct this field from today’s date, but all I get back in the field ID 53 is some date in 1970
I tried with and without quotes around $difference.
Thanks for your help
Hello Tobias, thanks for the reply. As you stated, you have our paid plugin could you please reach out to our support queue with this question so we could take a closer look into your setup? Go ahead log into your account here and submit a ticket. 😃
Is there a way to “translate” displayed date into a local language? My WP site is already using my language for dates but this snippet is displaying month and weekdays in English.
Any solution to this?
Thanks!
Hello Jernej, are you currently using a translation plugin? Is the plugin displaying the owords month and weekends in english or the actual month and weekend dates? Thanks!
Hello there, after contacting a developer regarding this, we actually do have an advanced version of this snippet available to Gravity Perk License holders. If you have a license could you please reach out to our support team and we would be more than happy to help out with this one.😃
Hi there, thanks for this useful article, I need to populate the target date field according to another date field (source) and a custom value which is called “nom” and holds the number of months (an integer)
target date field = source date field + nom months
for example: source date : 3/9/2020 nom : 10
the target must be as below: target date: 1/9/2021
the nom field ID is 14, and the other field numbers are mentioned in the following snippet code :
Configuration
$value = $entry[14]; new GW_Populate_Date( array( ‘form_id’ => 7, ‘target_field_id’ => 17, ‘source_field_id’ => 16, ‘modifier’ => ‘+$value months’ ) );
I retrieved the nom value using $entry[field_ID], but it still doesn’t work.
would you help me please?
In addition, isn’t it possible to calculate the target_date by AJAX at the time the user enters the nom value ?
best regards bro.
Hello Amin, Are you currently getting an error or is the nom value not displaying at all? Let us know and we can better assist!
Hi Ryan, how are you man? If I check the “required” option for the field and don’t select a date when submitting the form, it shows the common error that the field doesn’t have a value. But when I uncheck “required” option of the field, it doesn’t show an error, but after submitting the form, in the Inbox the NOM value is stored as “01/01/1970”. would you help me please?
Regards.
Hello Amin, Thanks for the description. We have a perk that is a better fit for this. It’s called Date Time Calculator and it would allow you to calculate/populate the user’s months with a calculation like {Date:1} – {today}. As for your issue, regarding the nom value being stored while unrequired, we would need to take a deeper dive into your form’s configuration to better assist with this one. We would be happy to dig into this via our support form. Thank you! 😀
Hi again, Isn’t it possible to help me here like other applicants please? because, to be honest, I’m using the free version of this module and therefore I will not be supported :( I’m so sorry <3
I believe the create_function() used in this snippet is depreciated.
Thanks for the extension, it works great. In the array I give at ‘modifer’ => ‘+18 months’, it’s okay, but i want a random date between 18,19 or 20 months or random between 547 – 608 days from the source field. What I need change? Thx for help & support.
Error message Uncaught Error: Class ‘GW_Populate_Date’ not found in ….. What could i be doing wrong?
This will help: https://gravitywiz.com/documentation/snippet-troubleshooting/
Hey, stumbled across this while trying to figure out how to convert the date format for admin. I couldn’t see a way to add a certain format to the admin dropdown, and I had read the only way to get a new date format was to use 3 fields with gform_pre_submission_filter to concatenate those fields together.
For the most part the snippet here works amazing! However, I get two entries each time a form is submitted (one with the current date and one with the newly formatted date).
I’m sure there’s probably a much simpler snippet I need, or just part of this snippet to get the outcome I’m going for.
Is there a way to only modify the date format entered by a user into a different format for admin within the same input field? Totally fine to use a hidden field for the newly formatted date as well if needed.
Thanks so much!
Mike
We don’t have a solution for this, Mike. Our recommendation would simply be to have two fields, one for the admin and one for the user.
Hi guys, is it possible to use this in a time field at all please?
Thanks
Ben
As in could I let the user select a time of day like 3pm and add and hour to another field to make 4pm please? This would be most useful.
This does not currently support Time fields. You could certainly do this if you were populating the time into a Single Line Text field though.
Hi there, thanks for this… is it possible to add a modified date into an html block using a shortcode?
Thanks,
Ben.
If you use this snippet to populate a field with the modified date, you could display that modified date in an HTML field with live merge tags (a feature of our Gravity Forms Populate Anything plugin).
Hey there :)
Was using this snippet successfully for a couple years, and now suddenly the date is being rejected by the form because it requires (mm/dd/yyyy) and what is being populated is (Month dd,yyyy) All the snippet parts in the functions file refer to m-d-y and even if i change it to MdY or any variation, the result remains the same. How the heck do I force the form field to populate the digits rather than formal name, and without the comma?
Hugely appreciate any help!
Hi David – the snippet is being rejected when I try to add it to functions.php
Is there a workaround or paid version of the snippet?
There are a few options: https://gravitywiz.com/documentation/how-do-i-install-a-snippet/
Hi David! Thanks for your quick response. I’m not sure why, but the snippet still isn’t working. When I attempt to use it, an error message pops up saying…
“Class ‘GW_Populate_Date’ not found”….followed by strings
oh my — silly mistake David, sorry! [slaps forehead] — you’ve been great! I have not. lol
Can this be used with some logic? I want to take a date and add a month, 3 months or a year based on the value of another field.
We have an advanced version of this snippet that supports field-based modifiers. In this way, you could create a field that had a numeric value for the number of months that should be added (1, 3, 12) and the date would be modified based on the user’s selection. The advanced version is available to Gravity Perks customers via support.
Can this be used to populate a field with the user’s age? I have a GF with an input for the user’s DOB and another field for their age. I’d like for the age field to be dynamically populated once the user enter’s their DOB.
Is that possible?
Hi Chris, we have an early-access perk that is a better fit for this. It’s called Date Time Calculator and it would allow you to calculate/populate the user’s age with a merge tag like {Date:1:age}. If you’re a Gravity Perks customer, we’ll be happy to send you a copy of this plugin via support.
Thanks David! Awesome product BTW!
My pleasure, Chris! Glad you like it. ?
Curious if this can be applied to labels – specifically those in lists. Say I wanted to get the state a person lived in over the last three years. I wouldnt want to change my form from 2017, 2016 and 2015 to be 2018, 2017 and 2016 when the new year rolls around. It would make more sense to have the list field column headers update based on the current year?
This isn’t a great fit for this use-case. I would recommend setting the List column labels via the gform_pre_render filter and PHP’s date() function to get the desired years.
The advanced version of this snippet seems not working in newest version Gravity Forms 2.4.2, F12 showing error like this:
“gravityforms.min.js?ver=2.4.2:1 Uncaught RangeError: Maximum call stack size exceeded at GFCalc.replaceFieldTags (gravityforms.min.js?ver=2.4.2:1) at GFCalc.runCalc (gravityforms.min.js?ver=2.4.2:1) at GFCalc.runCalcs (gravityforms.min.js?ver=2.4.2:1) at HTMLDocument. (gravityforms.min.js?ver=2.4.2:1) at HTMLDocument.dispatch (jquery.js?ver=1.12.4:3) at HTMLDocument.r.handle (jquery.js?ver=1.12.4:3) at Object.trigger (jquery.js?ver=1.12.4:3) at Object.a.event.trigger (jquery-migrate.min.js?ver=1.4.1:2) at HTMLDocument. (jquery.js?ver=1.12.4:3) at Function.each (jquery.js?ver=1.12.4:2)”
I have a similar error message.
My setup: Gravity Forms Version 2.4.5 Theme: GeneratePress Version: 2.2.1 WordPress 5.0.3
Hi – Seems like a really nice snippet. Need your help to better figure out the following aspects:
The earlier version of this snippet, “Populate date, one year from current date” supposedly works for all forms by default without specifying a form Id. Is there a way to make this new snippet work for all forms by default, if all of them are supposed to use the current date + 1 Year time for expiry date (user specified start/end date is not required in my case). Or, should I go with the previous version of this snippet, if it is not doable with this new version.
Quote: “Why would you need the expiration date? It’d be nice if you could let the user know when their membership expires in the confirmation message or a notification email.”
Does the above statement imply that this notification email regarding the expiration date can only be sent at the time of filling the form? Or is there a way to send these notification emails to the user closer to the expiry date as well, for example, for a 1-year subscription, sending these notification emails as reminders 30 days, 15 days, 1 day before expiry date, and one after the expiry date during the grace period (to make payment for renewal of subscription)?
One way, I can think of is creating separate administrative visibility fields with current date + 335 days for reminder 1, current date + 350 days for reminder 2, current date + 364 days for reminder 3, and current date + 380 days for the final grace period reminder. And then, setting up separate notification email reminders for each of these fields to be automatically sent to user, when current date matches these respective reminder dates (by enabling conditional logic in form notification settings) with the snippet: “send manual notifications with Gravity Forms”. However, I can’t find the date field in the conditional logic fields in Form Settings –> Notifications. Is this doable and does this make sense? Or is there another way to accomplish this objective of sending expiry reminder emails to users?
Need a bit more clarity here. For instance, if the user chooses to renew his annual subscription 10 days before the expiry date, in that case, his new subscription expiry date should be set accordingly (+ 1 year and 10 days from the date of renewal payment) as his previous subscription has not yet expired. How to accomplish this using this min_date parameter or otherwise?
Request you to kindly help me out here with your invaluable guidance. Many thanks in advance,
Best, Ambuj
Hi David – Any update on the above queries. Kindly let me know if this is doable with the available free snippets, and if not, which Gravity perk(s) do I need to purchase to accomplish the above objectives fully. Thanks a lot for your help.
Best, Ambuj
Hi Dave, I have added this Snippet “How to Populate and Modify Dates” to my themes functions.php file and it’s working perfectly!!! It looks like I need to take this one step further. I am going to need to set the number of days added to each of my date fields based on one of the drop down values in the form. Can you or someone on your team provide me with some sample code how this would be done? Would that code be placed at the end of the php file or does it need to go to another place in the program. I’m not a PHP programmer so sorry if I have to ask some very basic questions.
Thanks again!! Jon
Hi Jon, that is not possible with this version of the snippet. We have an advanced version available to Gravity Perks license holders that you can be requested via support.
I’m getting an error and my functions.php won’t update. Here’s what it says:
Your PHP code changes were rolled back due to an error on line 729 of file wp-content/themes/Avada-Child-Theme/functions.php. Please fix and try saving again.
syntax error, unexpected ‘new’ (T_NEW), expecting function (T_FUNCTION)
What does this mean? Consider myself a php novice, I can copy and paste ;).
Hi Jessica, this will help: https://gravitywiz.com/documentation/snippet-troubleshooting/
Hi
thanks for the toturial, it helped me a lot.
But for one fo my sites, I need to register 2 dates on 2 differents forms.
I tried to add it in the GW_Populate_Date(), but only one of the 2 dates is really registered.
I can I do ?
Thank you for this snippet…it is very useful. Could you please let me know how I could implement this on several forms (rather than just the one – mulitple form_id)
Thank you for your help! Jim
Great Job but That is Not Work with Multiple Forms if we have more then one form how useful that snippet
Hi,
How would it be possible to automatically update a date field from a number of days field each time the entry is modified?
Let say I have a form with : – date field = D – number of days field = d – comments field
D and d are specified by user at first submission of the form.
Then users can modify the entry by adding comments. Each time the entry is modified D=D+d
Hope it make sense :D
Thanks in advance,
Michael
Hi Michael, we have an advanced version of this snippet that is available to Gravity Perks users which supports modifying the date by a user-submitted value; however, this advanced version of this snippet would not automatically add days when the user adds a note to the entry. This would required further customization.
Does this snippet conflict with any of the other date-related perks or the (advanced) GP Date Time Calculator perk? JFYI – (I also have gravity view and gravity flow)? I am having some problems applying it (fatal error if applied etc). I use quite bit of custom code applied via the My Custom Functions (plugin) – all working bar this snippet, I was thinking maybe it was non unique function names ? due to use of other date related perks?
I’m not aware of any conflicts. Drop us a line via support with more details on the exact error you’re seeing and we’ll be happy to take a look.
Hi I would like ask some help with my code:
I would like to set my datepicker to this:
if the dates is prior from current date to greater than 90 days then show this hidden Category A field, Else if the dates is prior from current date to less than 90 days then show this hidden Category B field.
Can you help me run some codes regarding to Gform datepicker conditional logic?
Hi Dawn, we have an awesome plugin that can help with this. It’s Gravity Forms Limit Dates.
Hello. Can this snippet be used for the following:
User Selected Date Field: User enters a date Number of Days Field: User inputs a number (ex: 90) Calculation Result Field (in date format): This returns the date which is 90 days from the User Selected Date Field.
So for example, the user inputs the following:
User Selected Date Field: 01/01/17 Number of Days Field: 90
And the following is calculated and populated into the Calculation Result Field: 4/1/17
Thanks!
Hello I use the moments.js library. Code like this –
This code has saved me HOURS!! Thank you so much!! I need to tweak it for my form though but its a bit complex for me. Would anyone be able to help?
I’m making a contract generator, and i’ve got the above code fully working to add 6 months term onto a user defined date. However how would I in addition add a user defined amount of months into the modifier part of the code?
Hi Simon, we provide complimentary support for our snippets to our Gravity Perks users.
I have a form that I use “internally” to log new clients — including the duration of their contract (3 months, 6 months, 9 months or 1 year). I then have links to my email system, trello, etc to keep track of those clients.
However, I would like to set up a notification, in Gravity Forms, that goes to the staff person assigned to that client (which is a user inputted selection), to tell them that that client has started TODAY and that the client’s end date is (TODAY + 3 months) or (TODAY + 6 months) etc. I would like to calculate that end date, depending on what I have input as the client’s duration.
Is there a way to set this up so that a field such as ENDDATE is calculated as TODAY + X where X is determined by what value we chose in the DURATION field?
I hope that made sense…
Hi Patrick, it’s not documented, but it is supported. Here’s an example configuration you can use as a start: http://snippi.com/s/u3vtwkg
Fantastic, thanks for the quick response David!
Sorry David, one more clarification. In the snippet you have
‘inputId’ => 11, ‘modifier’ => ‘-{0} weeks’,
What is inputId? Is that the field that states how many months the contract is? or something else?
And if it is the field with the duration, does it somehow inform the modifier line? ie in this example, would field 11 automatically change the 0 in ‘-{0} weeks’, to a different value?
Apologies for what is probably a basic question — very new to this.
P
Input ID is the field/input ID of the field whose value will be used to replace the {0} in the modifier. The script handles all the details. You just configure it. :)
I followed from the reply to Patrick
Hi Patrick, it’s not documented, but it is supported. Here’s an example configuration you can use as a start: http://snippi.com/s/u3vtwkg
But it seems not working as intended.
I got a php warning.
Warning: strtotime() expects parameter 1 to be string, array given in /home/xxx/functions.php
it refer to this:
I got 1970 end date.
new GW_Populate_Date( array( ‘form_id’ => 1, // update to your form ID ‘target_field_id’ => 16, // update to the ID of the ‘End Date’ field ‘source_field_id’ => 15, // update to the ID of the ‘Start Date’ field //’modifier’ => ‘+364 day’, ‘format’ => ‘d/m/Y’, ‘modifier’ => array( ‘type’ => ‘field’, ‘inputId’ => 14, ‘modifier’ => ‘-{0} day’, ), ) );
May i know what’s wrong with my code? Totally understand inputID is from the X’s value.
By the way, the fieldId 14 is radio button.
This has been working on our site, but we’ve noticed a PHP error showing up:
PHP Warning: mktime() expects parameter 4 to be long, string given in
The line it specifically targets is $timestamp = mktime( 0, 0, 0, $month, $day, $year );
Any idea what might be wrong?
Hm, I’m not able to recreate but you can probably avoid this by changing that line to this:
$timestamp = mktime( 0, 0, 0, (int) $month, (int) $day, (int) $year );
hello, Excuse me I used this code. but my site is persian and date is in Shamsi format, this code does not work correctly. all of dates +28 days show 2017-06-18, both of 2 fields is in Shamsi format but the second when Populates is in Miladi, please help me. it is so important!
Hi Mahya, this is outside of what we can cover in free support. If you pick up a copy of Gravity Perks, we’ll be happy to take a look at this via our complimentary snippet support that comes with Gravity Perks.
Does this snippet still work?
I want to have the date pre-populate for today’s date.
Thanks.
You shouldn’t complicate matters with this snippet, gravity form has this functionality built into it’s core already. Just select the date field,click on ‘Advanced’ then use a date merge tag to populate this field. This only works after the form has been submitted though.
For populating the current date, I agree with Ojadua. You can set the default value of a field with the {date_mdy} merge tag. For more advanced date population, use this snippet.
I guess this liitle code is not what I am looking for. However do you have by any chance a code that will allow client select mutiple dates I have a form that provide registration for event that will run entire month. Clients can purchase ticket for entire month or select several dayes out of the month So I am looking to see if is there plugin that would allow this happenned. So my question is in arsenall of your tools do you have anything I can use.
Thank you
Currently, the only way to select multiple dates is to create multiple date fields. Selecting multiple dates from a single field is something I’ve considered for Gravity Forms Limit Dates but it has not been implemented yet.
Hi David! Is it possible to use the merge tag ? For example there is merge tag Months (from 1 to 12 months). You need to determine the date according to the formula – Input date + merge tag.
Hi David, I was wondering if GC has the ability to just have two fields for an expiry date (MM / YYYY) rather DD /MM/YYYY. Our Australian Medicare Card expiry dates only have MM/YYYY shown on them and I need to create a form to enable patients to place in their MM/YYYY.
Kind regards: Ross
Try using the drop down date format and then you can use this snippet to populate a default date and hide the day drop down with CSS.
Hi,
When I try to put date format as d/m/y (13/2/2017) I get an error.
What should be the proper date format if I want to populate a date like this?
Thanks
Hi Asaf, based on the demo, I would expect this to work. If you’re a Gravity Perks customer, we can help you via the support form.
Hello, Is it possible to frame the choice of dates? Example: possibility to choose a date only between 15 March and 15 November.
GP Limit Dates can do this really easily.
I am able to get the date to populate when submitting a new entry. However, I need it to update when I update the entry using Gravity View’s Edit Entry feature. Do you know if this is possible?
Hi Shannon, this is not currently possible out of the box with this snippet. This might be something that the Gravity View team would help you get working though. :)
Ok, Thank you David. I will contact Gravity View!
Hi David,
I love this snippet, it seems to do exactly what I need. Only question I have is how to “conditionally” populate the modifier field? You alluded to this being possible here (I think)
Well, I just found this out, but apparently you can pass a value like “+2 weekdays” to the modifier. This will only work with the onload and onsubmission formats. <<<<<<<
I need to be able to pass one of three values to the modifier – +30 days, +3 month, +1 year. This is based on a previous field selection (subscription period).
Any clues on how I go about doing this?
Oh, I probably forgot to mention that this date field populates a custom field on the backend – that’s why I can’t have multiple date fields – only one can be processed on the form is submitted. If that makes sense?
Hi David,
I have used the above code in our site. If i add the above code, my site is getting crashed. Can you help me to fix this? I have used “GWDayCount” already in our site to calculate total days.
thanks, Ronith
Hi Ronith, see if these tips help: https://gravitywiz.com/documentation/snippet-troubleshooting/ If you enable WP_DEBUG and see an error related to the perk, let me know what the full error message is.
Hi David,
I used the full code in fuctions.php file. Site is not getting crash now. But the source field is not working. Date is not populating in the field for +1 year. Please advise
Hi David,
Can you please help me to fix the above issue? waiting for your reply.
thanks, Ronith
Hi, David
Tried to use this snippet where I needed to have multiple source fields (3 to be exact) and wanted to display them on the same target field. I tried to adapt the troubleshooting tips but had no luck. With only one it’s working perfectly so I didn’t miss any step. But as soon as I try to adapt for 3, no good.
Example of what I used below (adapted from “Multiple Instances, Same Form”). I also tried “Many Different Forms, Same Settings” solution but no luck.
Help please?
Thank you!
Hi Hugo, what is your end goal? If you just need to copy multiple fields to a single field, you can use this snippet: https://gist.github.com/spivurno/7029518
Hi David, thanks for your reply. I wanted to show the user a table with their appointments scheduled but had 3 different date fields for 3 different rooms, which means that if the calendar no. 1 was the one used, the other 2 would show empty. So I created a field to merge all calendars into one final output. (all this using the old free Gravity View plugin). Managed to make it work by duplicating the code for GW_Populate_Date_1, GW_Populate_Date_2 and GW_Populate_Date_3. Probably not the ideal method but hey, it’s working :p But I’ll give that snippet a go just in case :) Thank you once again :)
My pleasure, Hugo. :)
Hi
Can this work in multisite with blog_id getting picked up as well as form and field is?
thanks
Brendan
You can wrap the init code in a conditional based on your blog ID. Something like this: http://snippi.com/s/1f2rgfd
Hi there…
This is great code. I’m trying to do something very similar to your demo and I’m stuck. Right now you have the demo using the GW Populate Date snippet with a single text field being modified by one day or one year from the current date. I need to have a user specified date populating a single text field with a modified date in a format that I would choose . The single text population option seems to be immediate and not just shown upon submission. Is that possible? Thanks so much.
Hi Jean, I have a more robust version of this snippet that supports front-end population that is available to Gravity Perks license holders. You can make the request via the support form.
Is there a way to have it calculate business days? This is exactly what I’m looking for, but would like to be able to skip the weekends.
Well, I just found this out, but apparently you can pass a value like “+2 weekdays” to the modifier. This will only work with the onload and onsubmission formats.
I would like the GW Populate Date to work on 4 different forms. They have been duplicated so the date field numbers are identical, but the form IDs are different. It works fine with the initial form:) How do I change the code to work with all four forms? Do I add the form numbers separated by commas like: new GW_Populate_Date( array( ‘form_id’ => 5, 6, 7, 8, ‘target_field_id’ => 56, ‘source_field_id’ => 24, ‘modifier’ => ‘-4 days’ ) ); or do I just duplicate the whole new GW_Populate_Date function for each form?
Hi Dave,
Yes, you would duplicate the GW_Populate_Date Class for each unique form and just change out the form_id parameter. Thanks!
Hi!
I tried using the code, but the target field is not updating. Any thoughts why?
Thanks!
This version of the snippet does not support populating the target field before submission. I have a version of this snippet available for Gravity Perks license holders that supports populating the target field on the frontend.
Hello, I tried this snippet and I get a white screen with a syntax error. So then I tried your troubleshooting link but still couldn’t get it to work. I can’t leave the site overnight as being a white screen with an error, so I have restored the original functions.php file. I also watched your how-to video and tried the debugging with my wp-config file too, all to no avail.
However, I took your raw snippet and I put it as-is in a PHP tester and it says “FATAL ERROR syntax error, unexpected ” . $value . ” (T_CONSTANT_ENCAPSED_STRING), expecting ‘,’ or ‘)’ on line number 66″
Number 66 is: add_filter(“gform_field_value_{$key}”, create_function( ”, ‘return ” . $value . ”;’ ) );
The whole chunk is this (just so you know):
*******So, do you know how to fix this so it will work? I would really love it if you do…. Please help. I don’t write php, so I have no clue what’s wrong here…
Thanks!*********
Are you downloading the snippet from the “raw” URL and not directly from the article?
Yes, I downloaded directly from the raw URL. I tried it twice. It gave me the same fatal error. Note, it is now not on my testing server anymore; it’s been migrated to my client’s host. Same problem though.
Hi there – thanks for this snippet. However, when I add the top code to my functions file I get an error and white screen:
http://prntscr.com/bdcluy
Any ideas?
Thanks for any help …
Neal
Try these troubleshooting tips: https://gravitywiz.com/documentation/snippet-troubleshooting/
I copied and pasted the snippet into my functions page. However, I can’t access my WP Dashboard anymore. My site isn’t even loading. Any idea on how to fix this? (or prevent it when I get the site up and running again?)
These instructions might help: https://gravitywiz.com/documentation/snippet-troubleshooting/
Hi, This is great, thanks – but we have 1yr, 2yr and up to 10yr memberships. Is that possible? sample code? Many, many thanks Paul
Yes, you can just create multiple instances of the class and populate the different fields with the different length memberships: https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
Hi David,
First, this is a great bit of code. Now a caveat – I’m primarily a designer, so bear with me on my lack of fluency with programming.
My question: This works great for single date ranges. Currently we use it for a 10-Day temporary liability policy. The coverage start date is entered in the form and it populates a pdf template with that start date and an expiration date set for 10 days later. Now, we want to add a second option to the form for a 7-Day policy as well.
The agent would fill out the form and check a box for one of the two policies (’10-Day’ or ‘7-Day’) – can I use this code to add the appropriate number of days depending on the variable choice? If so – can you show me how?
Thanks!
Tom
Hi Tom, you’ll want to create two different fields and use conditional logic to show the correct field based on the desired date range. You can then create multiple instances of this snippet; one for each field.
https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
David, great snippet. I’m in the same situation as Bob, and while your solution did work by creating two different fields w/ conditional logic to correctly populate a pdf, I have one more layer of complexity here. I’m displaying the entries to the user on the frontend of the site, and in order to sort the results by date, I need the populated date in a single field. Otherwise, I have two columns with the populated date based on the conditional logic.
Is there any way around this you can think of?
Thanks, Bryan
Hi Bryan, you might consider using this snippet to map the two separate Date fields to a single Hidden field on submission. This way you can use the Hidden field as the sorting field.
Thank you for this code, works great! We’ve been using it for a couple months and just discovered an odd behavior. On some browsers, when people access our site without using “www” in from of the domain name, the page is just white and says GW_Populate_Date. In most browsers the domain just forwards to http://www.domain.com, but Firefox and Chrome on Windows 8 get stuck. Any idea why that might happen? The site is http://www.allyhealth.net or http://allyhealth.net, if you want to test that out.
Thanks! John
Hi John, this is going to be a server configuration thing rather than an actual issue with the snippet. The one thing you might want to check on is that there is no excess whitespace between PHP tags in your theme’s functions.php file. Look for
?> (any number of spaces) <?php
.Thanks, David, you were right! That fixed it. Appreciate the help!
Thank you for this code as it was exactly what i needed and it seem to work great! I need to check out the rest of your offerings and thanks for providing your work and help on the site..
Glad to help, Rick!
I was wondering if any of the perks make it possible so that you can EDIT the SUBMITTED DATE of entry in the BACKEND? I have to export large number of entries daily or every couple of days so I use the date range. The problem I have is that many of the submissions need get updated as they had incorrect data but entries I am updating have been submitted from a week to over couple weeks so when I update them as there are quite a few they are out of the date range export to my CSV. So I need a way to change the SUBMITTED DATE to the current date I used to UPDATE and corrected the information as it fall into the Date Range when I do my export..
regards rick
Hi Rick, I don’t currently have a solution for this.
What is the best way to utilize this snippet to modify/populate multiple dates? I need to set two (possibly 3) dates based on a date entered by the user. I am setting a pre date, and at least one post date based on the date entered by the user.
Did you ever get a reply on this? I need to do the same thing and have exhausted my paths of inquiry.
You can create multiple instances of this snippet like so: https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
David
Wondered whether you can help me. When I paste the code to modify the date. I have got error on format for the date at line 132: ‘format’ => ‘F j, Y’) // i.e. March 10, 2015
Full lines below
// populate date one year from today and specify format new GW_Populate_Date( array( ‘form_id’ => 39, ‘target_field_id’ => 7, ‘modifier’ => ‘+1 year’ ‘format’ => ‘F j, Y’) // i.e. March 10, 2015 ) );
Can you help please
Many Thanks
Peter
Hi Peter, looks like you’re missing a comma between ‘+1 year’ and ‘format’:
'+1 year', 'format'
Thanks for posting this code, very useful! One question I don’t see covered: how do you set up the config with multiple forms? We have 3 forms that require moving the date forward 90 days for a free trial period. I have it working with this code:
Configuration
new GW_Populate_Date( array( ‘form_id’ => 30, ‘target_field_id’ => 48, ‘modifier’ => ‘+90 days’ ) );
new GW_Populate_Date( array( ‘form_id’ => 17, ‘target_field_id’ => 65, ‘modifier’ => ‘+90 days’ ) );
new GW_Populate_Date( array( ‘form_id’ => 40, ‘target_field_id’ => 63, ‘modifier’ => ‘+90 days’ ) );
Which seems to work fine, but is that the best way to do it, or even right? I’m a newbie with the PHP, so could use a little help on this.
Thank you!
John
Hi John, that’s exactly how you do it. Good work!
How do you do this with the same form? I have 2 different variables in a single which require different expiration dates populated into a hidden field on ‘submit’.
This might help: https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
Hello,
At the bottom of the provided url you can see the date fiel using snippet to prepropulate field.
Unfortuntely the populated date is tomorrow, I wonder what causes the problem. What would you recommand ?… Thanks for your answer
Can you share a link to a pastie with your configuration of the snippet?
Hi, Everything seems to be quite normal now, date in form is actual date. I’ll get back to you if problem reappears, but for now it’s fine!
Dave,
I am looking at combining this snippet with your conditional date perk, but I have a very specific use case.
The plan is to build a lunch order form that would allow customers to place orders for days of the week.
Monday (date) Items available to order for Monday Tuesday (date) Items available to order for Tuesday Wednesday (date) Items available to order for Wednesday Thursday (date) Items available to order for Thursday Friday (date) Items available to order for Friday Submit
What I am looking to solve here is that orders for a specific day will close at noon the previous day.
So you would need to place an order before Thursday noon in the Friday section.
Any thoughts on this?
Do you have a URL where I could view your form? It’ll help me better understand what you’re trying to accomplish.
What if I wanted to fill the modifier in with the value from a field in the form?
So purely as an example:
We have source field (due date), target field (field that is calculated) and modifier field (urgency). The urgency values can be set to ‘+1 day’ or ‘+ 2 days’….etc….
I just cant figure out how to get the value from that field into the code you posted (I have it working as you intended, I just need to figure out how to modify it)
new GW_Populate_Date( array( ‘form_id’ => 1, ‘target_field_id’ => 31, ‘source_field_id’ => 9, ‘modifier’ => ‘+2 day’ (some how make this equal to the value of the modifier field(urgency) ) );
Any thoughts?
Thanks,
Jason Burns
Hi Jason, you’d need to modify the get_modified_date() method to fetch the field value that should be used to modify the date. You can get the submitted value via rgpost( ‘input_1’ ); replacing the “1” with the ID of your field.
Hi Jason,
have you figured out how this can be achieved? I can’t get it working, so I would be glad if you could give me some tips.
Hi Marian, if Jason doesn’t have a solution and you’re interested in hiring me to enhance this snippet with the necessary functionality, please do get in touch.
A nice perk, again :D !
Just a little question: I would really need a second date field to have the date of the first date field as a ‘minimum’ (it is about booking a flight + return flight), so already before someone clicks the ‘submit’ button. Is this somehow possible?
Also, just like Jesper, I would need both date fields not to have the possibility to choose a date in the past (although, if my first problem is solved, automatically the second date field wouldn’t be able to have this issue). Unfortunately, also for me the code you sent did not work. Would you have another solution for this?
Thank so much, again, in advance!
Regards from Amsterdam,
Johan
Hi Johan, I’ve just released GP Limit Dates which allows you to link date fields (i.e. set the min date on one field based on the selected date in another) and also to prevent days from being selected in the past (or future). Here are two articles that cover these topics directly.
This gave me a fatal error:
Class ‘GW_Populate_Date’ not found in …./functions.php
Hi Matt, did you include the full snippet and not just the initialization bit?
How can I make the date I choose in one date field ‘the arrival date’ display as one day later in another date field, i.e. the ‘departure date’?
Let’s say I put in April 1st as the arrival date, how can I have it automatically populate the departure date as +1 of whatever date I choose in the arrival date?
Hi James, I’ve just released GP Limit Dates which allows you to link date fields as you’ve described. Here are is an article that shows how easy it is to configure.
https://gravitywiz.com/how-to-restrict-dates-in-second-date-field-based-on-date-selected-in-first-date-field-with-gravity-forms/
Perfect, works a charm. Thank you!
Thanks Nick! Glad it helped you!
hi . it is very good post . but i want to change price field based on the current date or a user-submitted date. please help me .
Would be possible to populate many (or all of the) date fields, in many forms? I have to replicate the exact same form, and i need they to save a submission’s date, auto-populated and not visible for user (im thinking of hiding it vía CSS).
Thanks in advance, Nico
Hi Nicolas, the submission date is already stored as the Entry Date. Available via merge tag like so:
{entry:date_created}
.Thanks David!
Hey Jordan! Just integrated this snippet into one of my forms and it works great. Showing the date of today. But how to make the date field “not possible” to choose a day in the past? Do you have any “snippets” for that?
Hi Jesper, here’s a snippet you can add to an HTML field on your form: http://pastie.org/private/rcrifox4toaduvblixa
Replace the “12” with your form ID and the “8” with your field ID.
Hi David, I have tried to paste the code in my form in a html field with no result,0 only the code was visible in frontend? I did change the IDs. I am not sure if am doing right … and how to make the code not visible?
Hi Jesper, I’ve just released GP Limit Dates which supports this without any code required. Here’s a How To article answering your specific question.
https://gravitywiz.com/how-to-require-a-future-or-past-date-with-gravity-forms-date-picker/
I am trying to create a timesheet form. Person selects a pay period from a drop down, the form auto fills the dates (starting with the pay period date selected), the user then fills in their time and it is submitted.
I used a list for the day, date, time, and type of hours.
Is there a way to key the date to the drop down to auto populate?
I think that many small business owners with a handful of employees would find such functionality helpful.
Hi Ellie, do you have a URL to a page where I could see your setup?
Thanks David – Correct, trying to use this based on user submission, and no, unfortunately it’s not being populated even after submission. :( Target field starts, and ends, as blank, and I’m not sure how to troubleshoot it any further.
Hi there, first off thanks for the wonderful site, it’s a treasure trove of useful information! I love the idea of this snippet, I just can’t get it to work so far and am not sure where I’m going wrong. After digging around in the previous tutorial, I’m wondering if it has to do with the parameters of the target field.
Right now my target field is a ‘Single Line Text’ field, with the ‘gf_hidden’ class applied, and in the field’s advanced properties I checked ‘Allow field to be populated dynamically’ and tried using a parameter name of both Populate_Date and GW_Populate_Date. So far no luck getting it to fill in the modified date.
The configuration I’m using is…
new GW_Populate_Date( array(
) );
And I’ve triple-checked that all the field IDs (and the form ID) are correct.
Help?? And thank you again for the awesome resource!
If you’re using the source_field_id parameter (aka the snippet is based on user input), the date won’t be populated into the target field until you’ve submitted the form. Is it not being populated even on submission?
Also, just a heads up, you don’t need to enable the dynamic population for this field. This snippet does not use that technique.
Hello,
i would love to use this to display when my customer’s orders will be ready. However, I have been unable to get this to work – are there more specific instructions (for ‘dummies’)? I have pasted the code in, but unclear on what to change or how to enter it into a form. I am just looking for an HTML field to display the date (no customer input, just display 30 days from today).
Thank you!
Even though the customer won’t interact with your +30 days date, you will still need to add a Date field to the form so that the snippet can populate it with the +30 days date. You can then hide this Date field by adding “gf_hidden” to your field’s “CSS Class Name” setting. Assuming your field ID was “3” and your form ID was “1”, your config would look like this:
new GW_Populate_Date( array( 'form_id' => 1, 'target_field_id' => 3, 'modifier' => '+30 days' ) );
I am running into an issue where the date does populate correctly (yay!) but does not map over correctly to Salesforce for some reason… This is a long shot, but does anyone know why the date is not mapping over to Salesforce like the other form fields?
It actually doesn’t map over at all and prevents the whole form from mapping over to the other fields…
Replied to your email. You are most likely correct with your order-of-events theory.
I have 3 different forms that need to populate an expiration date one year from the date the form is submitted. For the code to work properly with all 3 forms, can I just add each form ID followed by a comma after the ‘form_id’ => ? Please let me know! This has been a lifesaver!
Hi Dave, this might help clarify: https://gravitywiz.com/documentation/apply-class-based-snippet-different-forms/
Is there a way to restrict users from selecting dates within a certain time frame?
What I need to do is limit/restrict the date range so only dates 48 hours or later from the data of submission can be selected. But I haven’t seen a simple snippet to do this.
Hi Dakota, your comment was lost. Sorry about that. This is now possible via GP Limit Dates.
Just came across this snippet, and it’s exactly what I am looking for. Although I can’t get it to work. I am running the latest version of GF and WP, and I am trying to Populate Date One Day from User-specified Date by inserting this into my theme’s functions.php:
new GW_Populate_Date( array( 'form_id' => 1, 'target_field_id' => 2, 'source_field_id' => 1, 'modifier' => '+1 day' ) );
Although I keep getting this:
Fatal error: Class ‘GW_Populate_Date’ not found in /functions.php
This is great for a default on how long submitted ads should run – user sets the start date and the end date is automatically a week later. Beautiful!
Hi David,
Thanks for re-directing me to this new code and it does work but I am still get a date of 1970. Is this something with my server pulling the wrong date or a setting somewhere? My wordpress is showing the correct date and time in the settings so I am not sure where to look.
Thanks Steve
I narrowed down my issue to it just needing to be in a epoch/unix format. My plugin uses this format to determine expire date. How can I get the output from this code into the correct format?
Example: 1447534155 = Sat Nov 14 15:49:15 2015
Thanks
Hey Jordan! Just integrated this snippet into one of my forms and it works great. I used it for one of my forms where you have to send the date that funds are set to clear to a third party. So, as funds that go through to the third party clear 3 days from whenever the transaction takes place, I set it to + 3 days and it works like a charm. Love the stuff you and David are coming up with, it makes Gravity Forms so much better!
Thanks Catharine! We’re glad you’re finding it useful.
Hi, Nice and Awesome tweaks for eagerly having from long time. Thanks for this. It would be better to add some jquery to populate in form. Would rather better if it displayed in form before submit.
Thank you and your team from heart.